This is sample application to serializes sample object
using System;
using System.Collections.Generic;
using System.Text;
using System.IO;
using System.Xml.Serialization;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
SampleObject sampleObject = new SampleObject();
sampleObject.Id = 35;
sampleObject.Name = "Ozan K. BAYRAM";
XmlSerializer serializer2 = new XmlSerializer(typeof(SampleObject));
MemoryStream ms = new MemoryStream();
XmlSerializerNamespaces xmlSerializerNamespaces = new XmlSerializerNamespaces();
xmlSerializerNamespaces.Add("", ""); //if you want namespace for your XML define here
serializer2.Serialize(ms, sampleObject, xmlSerializerNamespaces);
StringBuilder XMLText = new StringBuilder();
ms.Position = 0;
using (StreamReader Reader = new StreamReader(ms))
{
XMLText.Append(Reader.ReadToEnd());
}
string serializedObject = XMLText.ToString();
}
}
}
Output string:
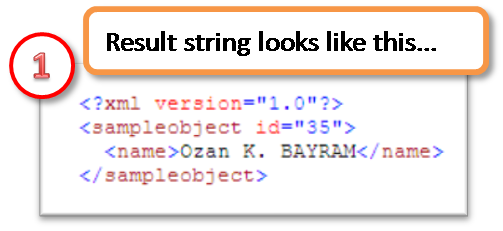
Sample class to use:
[Serializable]
[System.Xml.Serialization.XmlRoot("sampleobject")]
public class SampleObject
{
[System.Xml.Serialization.XmlAttribute("id")]
public int Id { get; set; }
[System.Xml.Serialization.XmlElement("name")]
public string Name { get; set; }
}